When using the built-in color convert function in opencv to convert a RGB image to grayscale, the result may not be good, as the intensity information are lost. Inspired by Poisson Blending, we can convert the image to HSV first, and then treat the saturation channel as source image and value channel as target image, so basically we are copying S channel to V channel and the result will be a grayscale image. Apparently, the results can be much better.
Indicated by our experiments, however, the result can be too "bright", where the values exceed 255. Therefore, a darker version will also be generated by brutely reducing 50% light intensity, to get a better output.
Input | cv2 | Original Output | Darker Version |
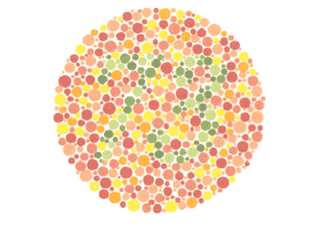 |
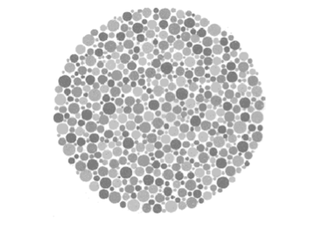 |
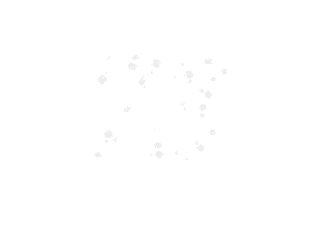 |
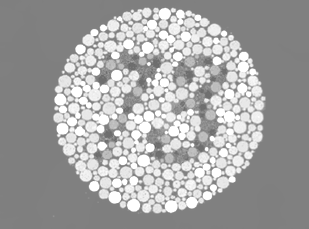 |
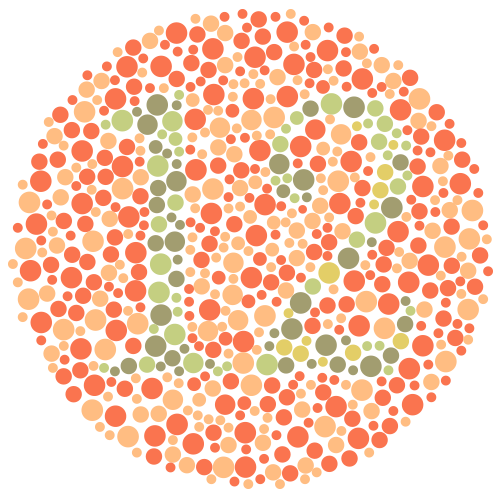 |
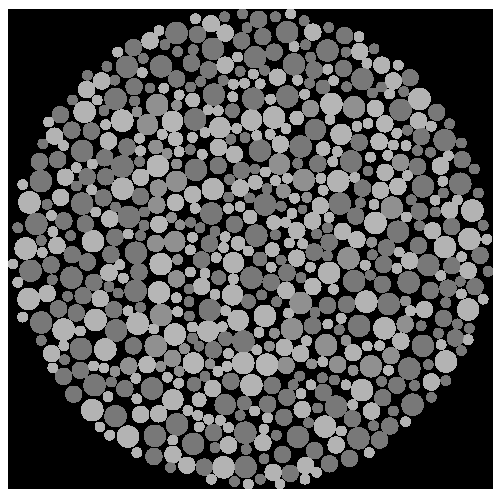 |
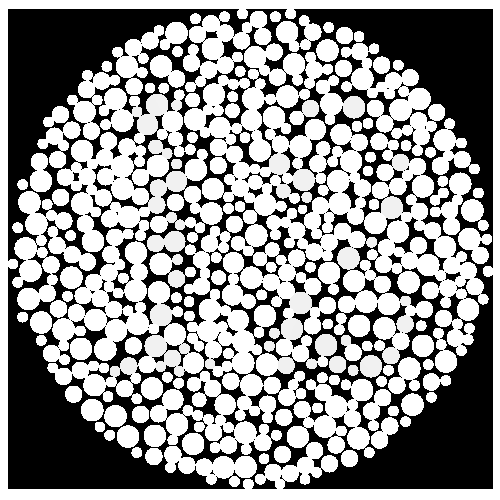 |
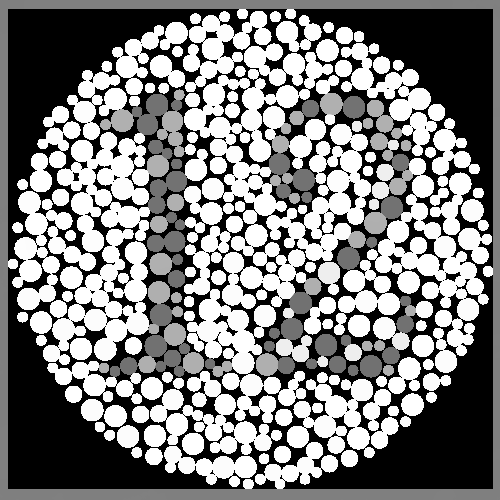 |
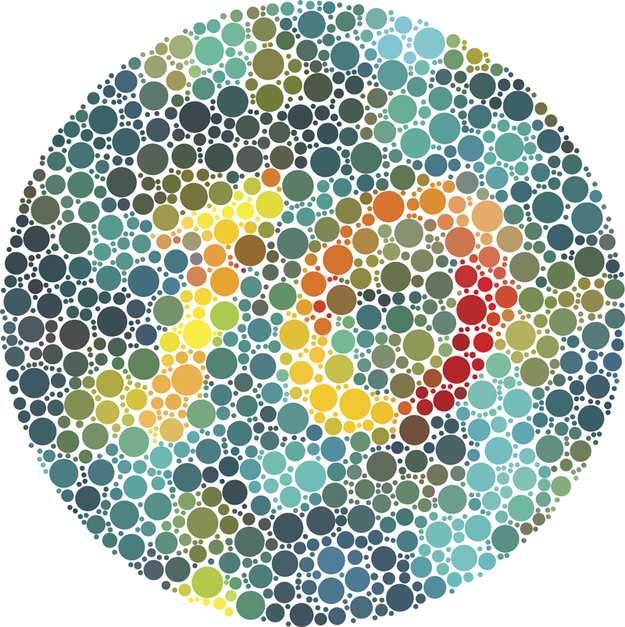 |
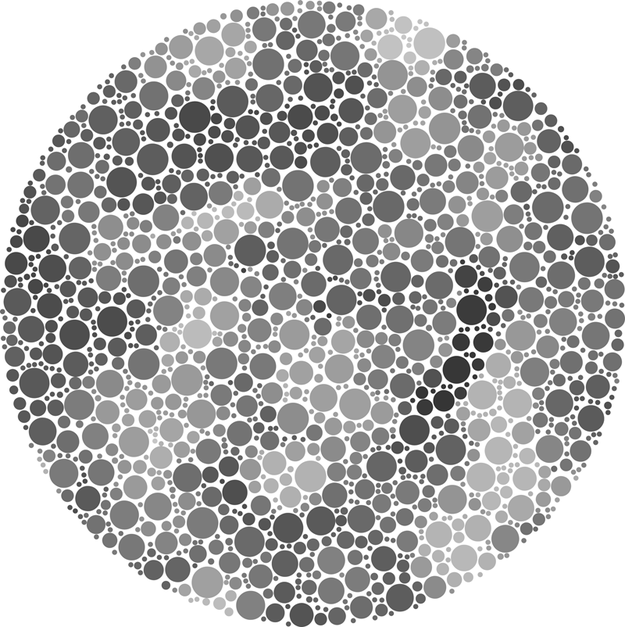 |
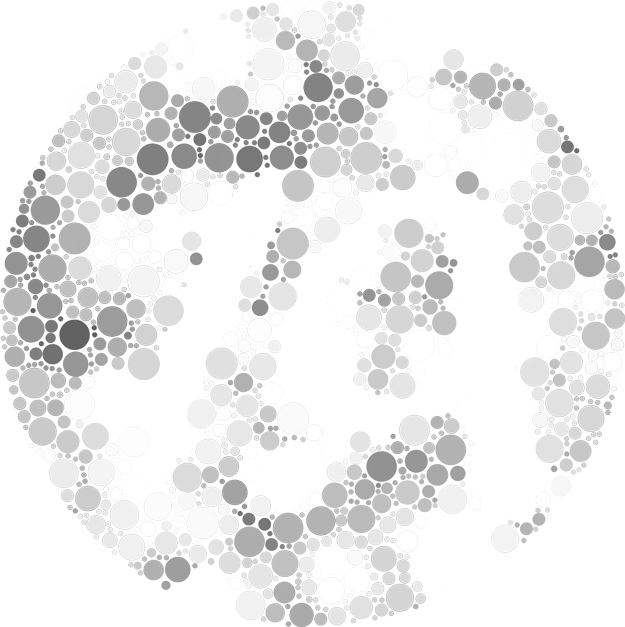 |
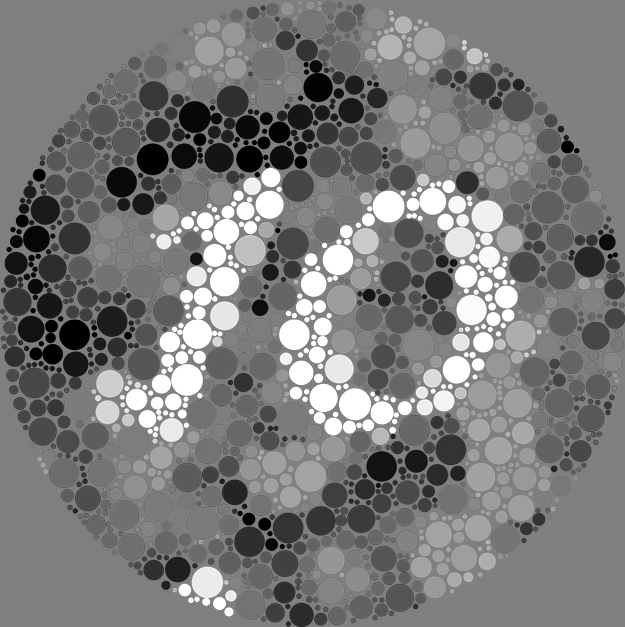 |
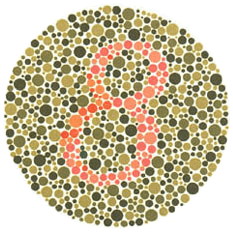 |
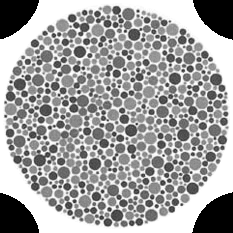 |
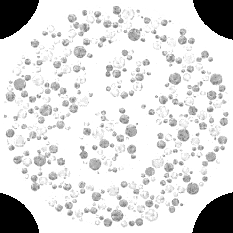 |
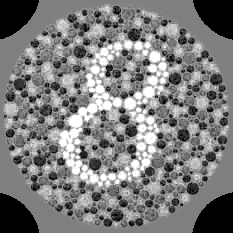 |